Nati's Blog
Web Application Performance
Introduction
In today's competitive digital landscape, app performance can make or break user experience. Users expect applications to be responsive, fast-loading, and smooth—regardless of device or network conditions. Studies consistently show the business impact of performance:
- A 100-400ms delay in app response can reduce user engagement by 0.2-0.6%
- A 1-second performance improvement can increase conversions by up to 27%
- 53% of mobile users abandon sites that take longer than 3 seconds to load
This guide explores proven strategies to optimize your app's performance, complete with practical implementation APIs that offer free tiers or open-source options.
Understanding App Performance Fundamentals
Before diving into optimization techniques, let's clarify key performance metrics:
- Latency: The delay between a user action and the app's response
- Time to Interactive (TTI): How long before users can interact with your app
- First Contentful Paint (FCP): Time until the first content appears
- Memory Usage: How efficiently your app uses device RAM
- CPU Utilization: Processing power required by your app
Performance bottlenecks can occur in multiple places:
- Network Layer: Slow data transfer between client and server
- Server Processing: Backend delays in generating responses
- Client-Side Rendering: Frontend slowness in displaying content
- Resource Consumption: Inefficient use of device resources
Essential App Performance Optimization Strategies
Let's explore eight core strategies to dramatically improve your app's performance, along with APIs to help implement them.
1. Efficient Data Loading and Caching
Perhaps the most fundamental performance optimization is minimizing network requests by implementing robust caching.
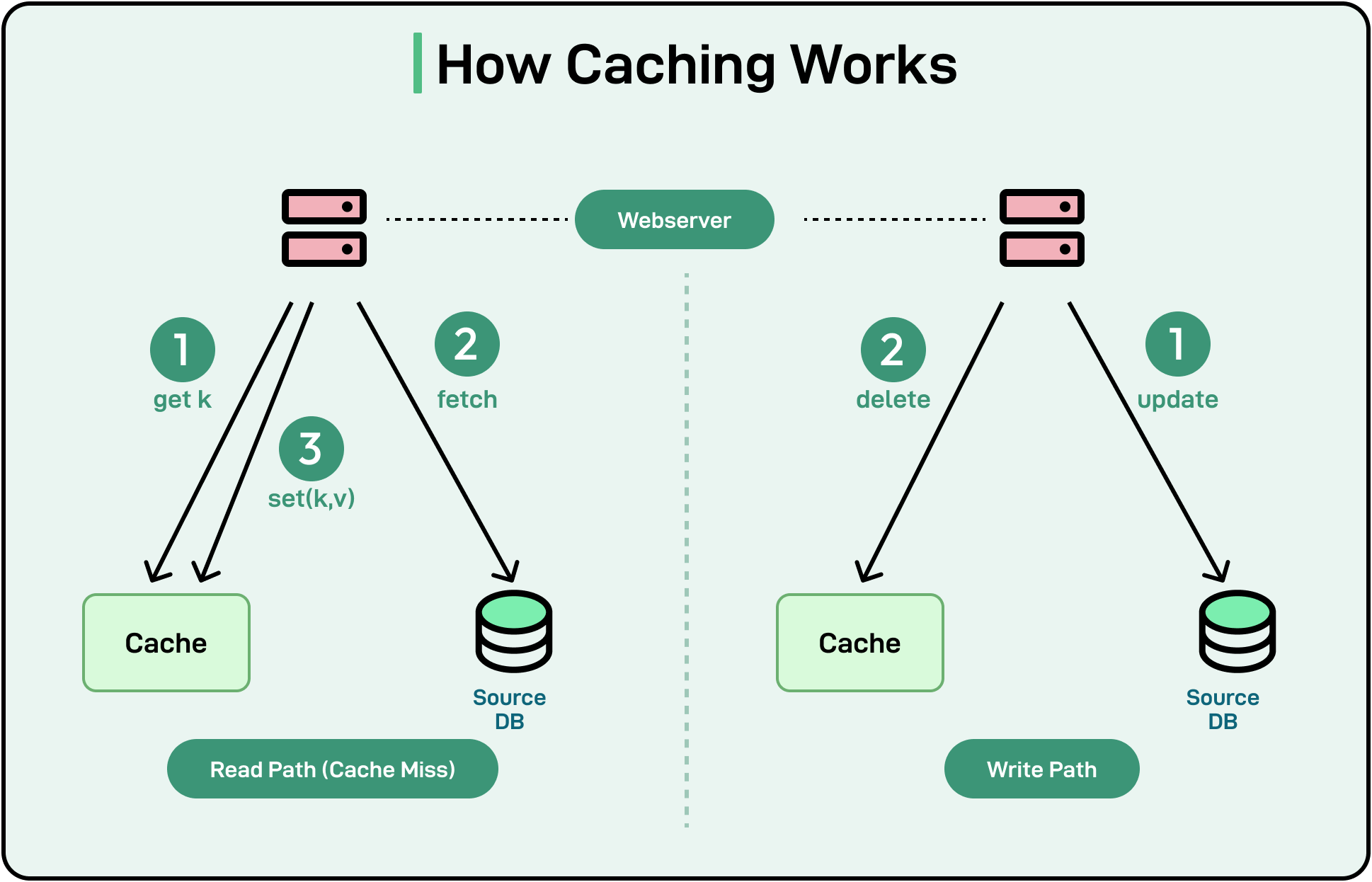
Implementation: AppsFlyer Cache API
AppsFlyer provides a powerful mobile SDK with caching capabilities for apps.
Example integration:
// Initialize cache with 10MB limit and 24-hour TTL const cache = new AppsFlyerCache({ maxSize: 10 * 1024 * 1024, // 10MB defaultTTL: 24 * 60 * 60 * 1000 // 24 hours }); // Store user profile data async function cacheUserProfile(userId, profileData) { await cache.set(`user:${userId}:profile`, JSON.stringify(profileData)); } // Retrieve from cache with fallback async function getUserProfile(userId) { const cachedProfile = await cache.get(`user:${userId}:profile`); if (cachedProfile) { return JSON.parse(cachedProfile); } // Cache miss - fetch from server const profileData = await fetchProfileFromServer(userId); // Store in cache for next time cacheUserProfile(userId, profileData); return profileData; }
Benefits:
- Reduces server load and network requests
- Improves app responsiveness with instant data access
- Works offline for previously cached content
Free tier: Available with AppsFlyer's free plan Documentation: AppsFlyer SDK Documentation
2. Image and Media Optimization
Media content is typically the heaviest resource in mobile apps. Optimizing images and videos can dramatically improve load times.
Implementation: Cloudinary API
Cloudinary offers comprehensive media optimization through their mobile SDKs and APIs.
Example Postman request to optimize app images:
POST https://api.cloudinary.com/v1_1/{your-cloud-name}/image/upload Headers: Authorization: Basic {base64_encoded_credentials} Content-Type: application/json Body: { "file": "https://your-app-resources.com/original-image.jpg", "eager": [ { "width": 750, "height": 422, "crop": "fill", "quality": "auto:good", "format": "auto" }, { "width": 375, "height": 211, "crop": "fill", "quality": "auto:good", "format": "auto" } ], "eager_async": true }
This request creates two optimized versions of your image: one for regular displays and a smaller one for thumbnails.
Mobile SDK integration:
// Android example CloudinaryMedia mediaManager = new CloudinaryMedia(context, "your-cloud-name"); // Load optimized image into ImageView mediaManager.loadImage("product_id_123.jpg") .resize(width, height) .quality("auto:good") .format("webp") .into(productImageView);
Benefits:
- Automatic format conversion to WebP/AVIF
- Responsive images sized appropriately for devices
- Lazy loading and progressive rendering
- Bandwidth detection and quality adjustment
Free tier: 25GB storage, 25GB bandwidth, 25,000 transformations monthly Documentation: Cloudinary Mobile SDK
3. Network Optimization
Efficient network communication is crucial for app performance, especially on mobile networks with varying conditions.
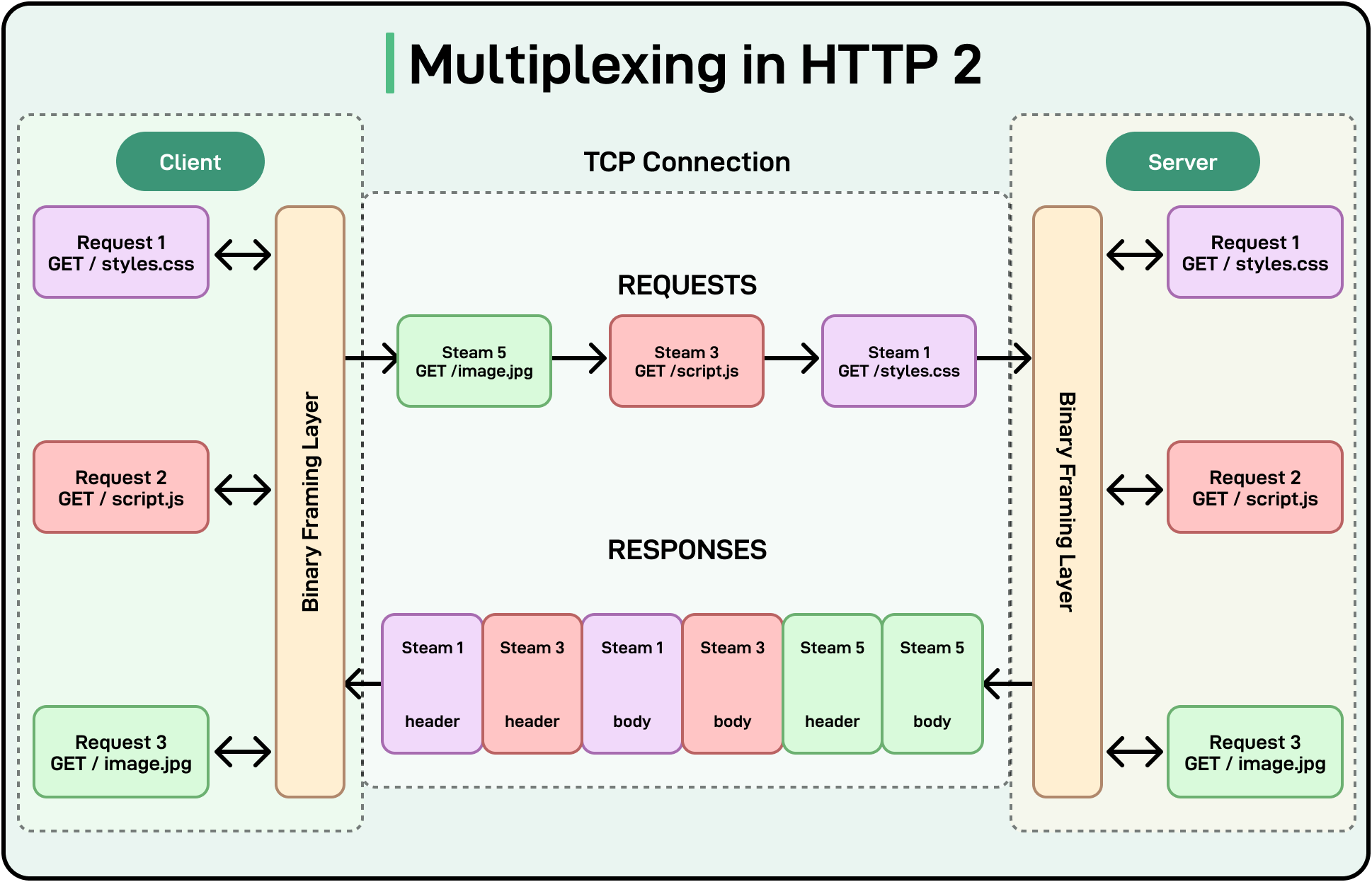
Implementation: Gzip Compression with Retrofit
For Android applications, Retrofit with OkHttp provides efficient network communication with built-in compression.
Example implementation:
// Configure OkHttp client with compression OkHttpClient client = new OkHttpClient.Builder() .addInterceptor(new GzipRequestInterceptor()) .build(); // Create Retrofit instance with the client Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .client(client) .build(); // GzipRequestInterceptor implementation public class GzipRequestInterceptor implements Interceptor { @Override public Response intercept(Chain chain) throws IOException { Request originalRequest = chain.request(); // Don't compress if already compressed if (originalRequest.body() == null || originalRequest.header("Content-Encoding") != null) { return chain.proceed(originalRequest); } // Compress the request body Request compressedRequest = originalRequest.newBuilder() .header("Content-Encoding", "gzip") .method(originalRequest.method(), forceContentLength(gzip(originalRequest.body()))) .build(); return chain.proceed(compressedRequest); } // Implementation details omitted for brevity }
Benefits:
- Reduces data transfer size by 70-90%
- Decreases battery consumption on mobile devices
- Improves app responsiveness on slow networks
- Minimizes data usage for users on metered connections
Free tier: Open-source libraries with no usage limits Documentation: OkHttp and Retrofit
4. Content Delivery Networks (CDNs)
CDNs distribute your app's static resources across global servers to reduce latency and improve download speeds.
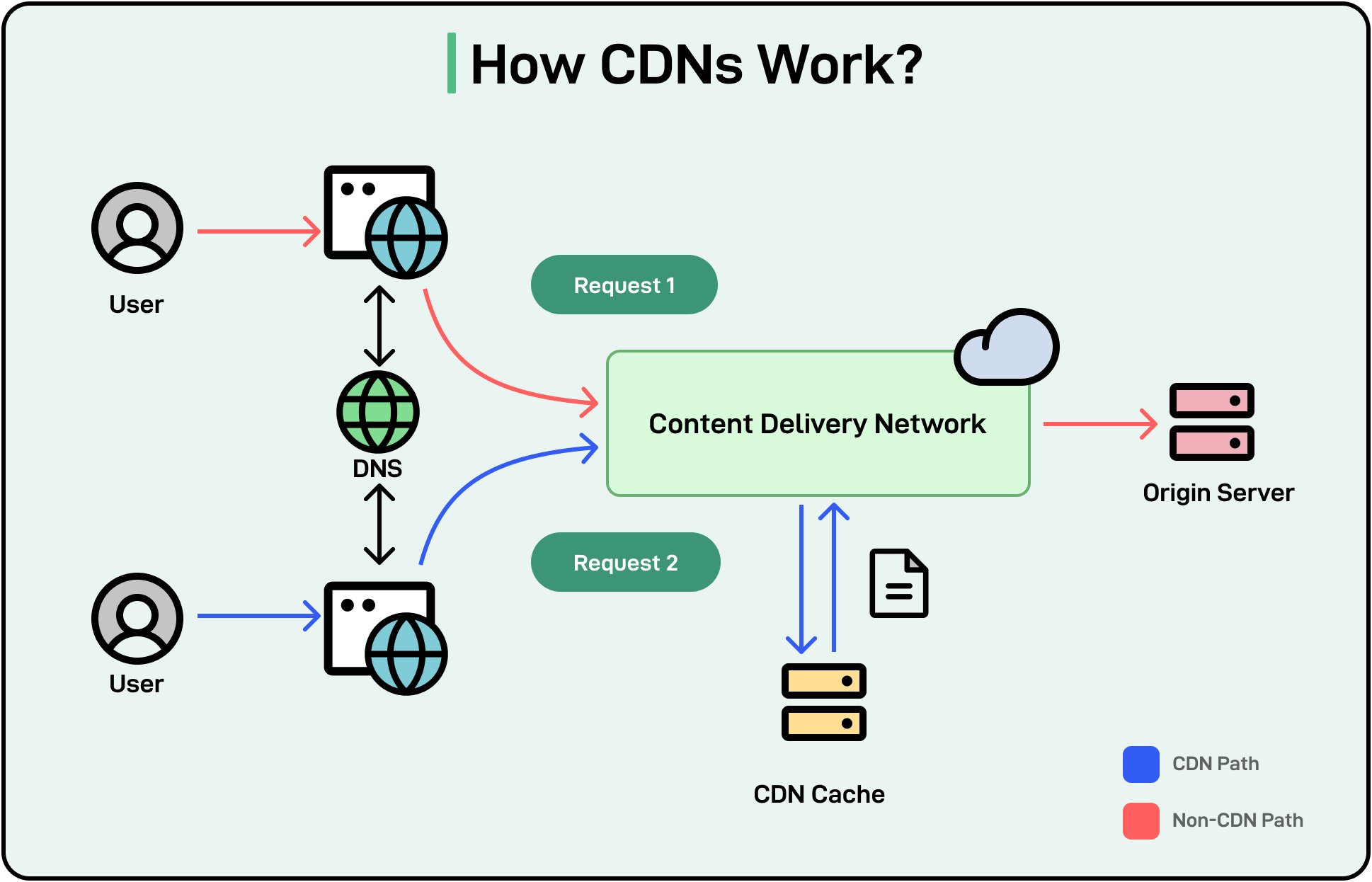
Implementation: Fastly CDN API
Fastly provides a powerful CDN with an API for managing content distribution.
Example Postman request to purge cache:
POST https://api.fastly.com/service/{service_id}/purge/{resource_id}
Headers:
Fastly-Key: {your_api_key}
Accept: application/json
Integration in mobile apps:
// iOS example using URLSession with CDN let configuration = URLSessionConfiguration.default configuration.requestCachePolicy = .reloadRevalidatingCacheData configuration.urlCache = URLCache(memoryCapacity: 10 * 1024 * 1024, diskCapacity: 50 * 1024 * 1024, diskPath: "fastlyCache") let session = URLSession(configuration: configuration) // Request assets from CDN let url = URL(string: "https://cdn.fastly.example.com/assets/app-logo.png")! let task = session.dataTask(with: url) { data, response, error in if let data = data { DispatchQueue.main.async { self.logoImageView.image = UIImage(data: data) } } } task.resume()
Benefits:
- Reduces app startup time with faster resource loading
- Improves reliability during traffic spikes
- Provides edge computing capabilities for dynamic content
- Offers DDoS protection and security features
Free tier: Developer testing accounts available Documentation: Fastly API Reference
5. Database Performance Optimization
Database operations often create bottlenecks in app performance, especially for data-intensive applications.
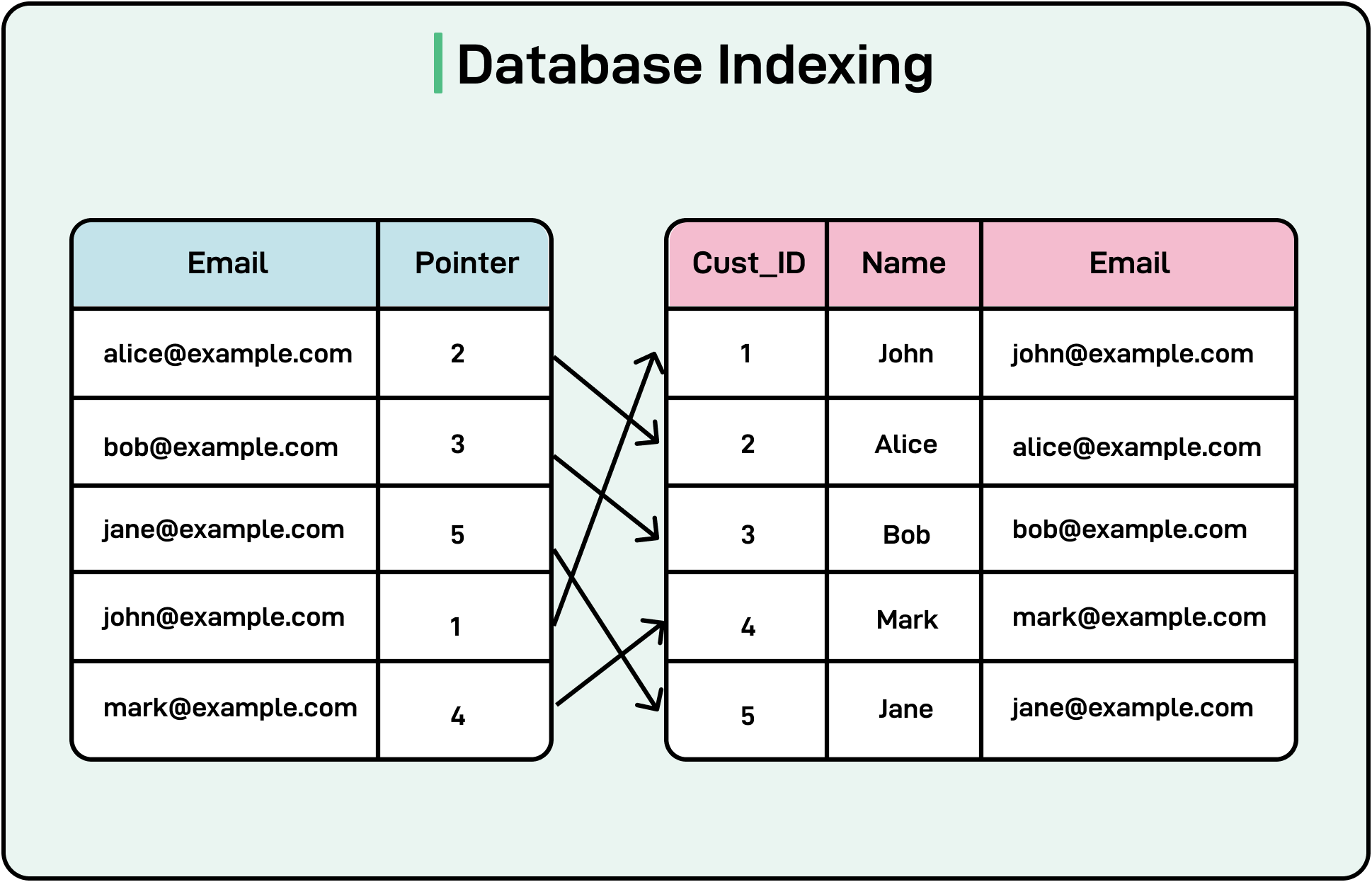
Implementation: Realm Mobile Database
Realm provides a high-performance mobile database optimized for app development.
Example implementation:
// Define your data model public class Product extends RealmObject { @PrimaryKey private String id; private String name; private double price; private String description; private int stock; // Indexing frequently queried fields @Index private String category; // Getters and setters } // Initialize Realm Realm.init(context); RealmConfiguration config = new RealmConfiguration.Builder() .schemaVersion(1) .build(); Realm realm = Realm.getInstance(config); // Query with indexing RealmResults<Product> electronicsProducts = realm.where(Product.class) .equalTo("category", "electronics") .sort("price") .findAllAsync();
Benefits:
- Significantly faster than SQLite for mobile apps
- Zero-copy architecture minimizes memory usage
- Offline-first design with automatic synchronization
- Reactive architecture for responsive UIs
Free tier: Free for mobile app development Documentation: Realm Database Documentation
6. Load Balancing for Backend Services
For apps that rely on backend services, load balancing ensures consistent performance during usage spikes.
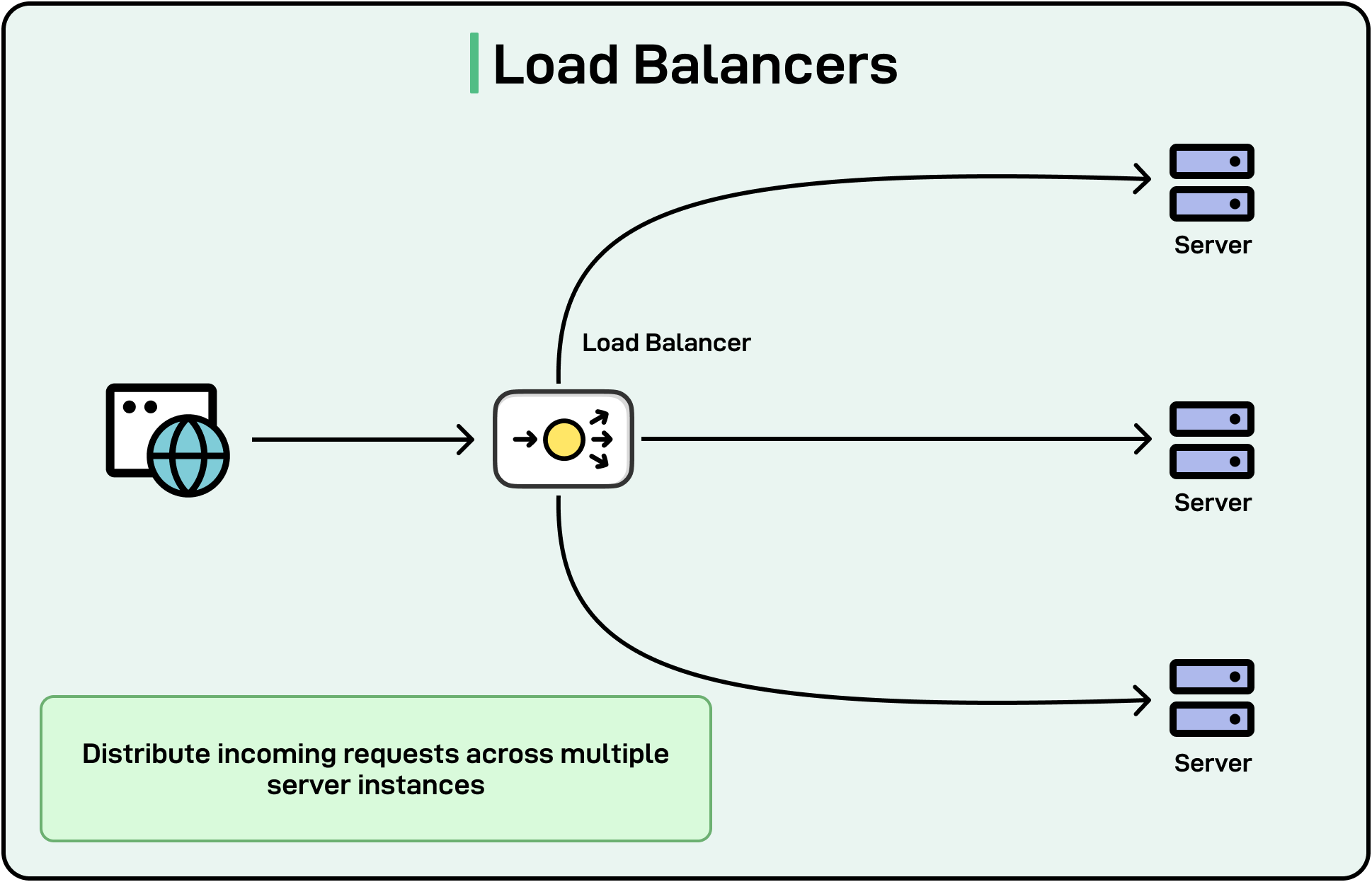
Implementation: Firebase App Distribution
Firebase offers app distribution with built-in load balancing for backend services.
Example setup in Firebase console:
- Configure auto-scaling in Firebase Hosting
- Set up Cloud Functions with appropriate memory and instance settings
- Enable Firebase Performance Monitoring to identify bottlenecks
Integration in your app:
// Initialize Firebase Performance Monitoring import { getPerformance } from "firebase/performance"; const perf = getPerformance(); // Track custom traces for critical operations async function loadUserData() { // Start performance trace const trace = perf.trace("user_data_load"); trace.start(); try { // Perform data loading operation const userData = await fetchUserDataFromBalancedBackend(); return userData; } finally { // End trace trace.stop(); } }
Benefits:
- Automatic scaling during usage spikes
- Global distribution of backend services
- Built-in performance monitoring
- Seamless failover for high availability
Free tier: Generous free tier with Firebase Spark plan Documentation: Firebase Documentation
7. Asynchronous Processing and Background Tasks
Moving intensive operations off the main thread improves app responsiveness and prevents UI freezing.
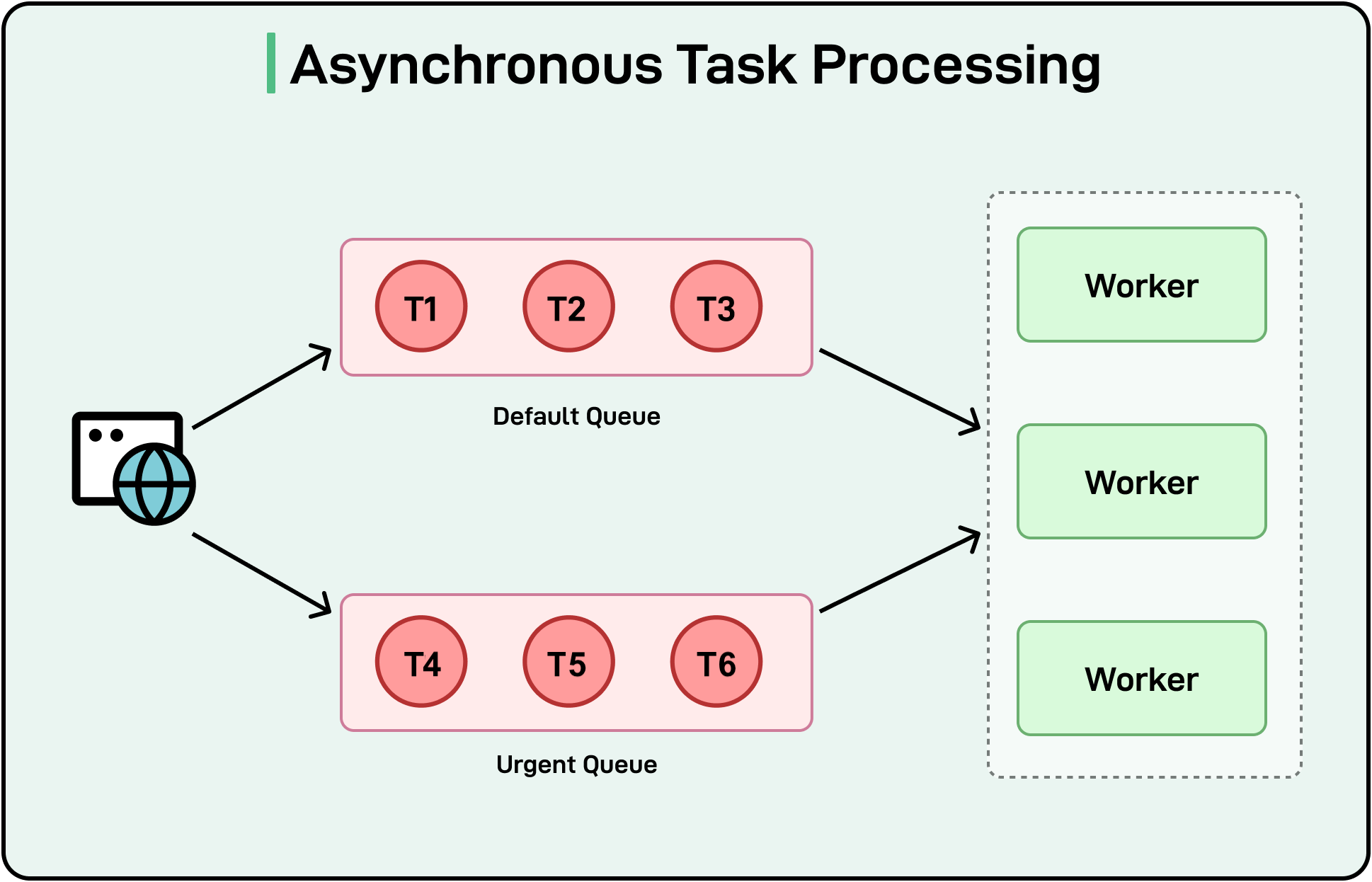
Implementation: WorkManager API (Android)
WorkManager provides a robust API for handling background tasks in Android applications.
Example implementation:
// Define a background work task public class ImageProcessingWorker extends Worker { public ImageProcessingWorker(Context context, WorkerParameters params) { super(context, params); } @Override public Result doWork() { // Get input data String imageUri = getInputData().getString("IMAGE_URI"); // Process image in background boolean success = processImage(imageUri); // Return result if (success) { return Result.success(); } else { return Result.retry(); } } private boolean processImage(String imageUri) { // Image processing logic // ... return true; } } // Schedule the work Data inputData = new Data.Builder() .putString("IMAGE_URI", "content://media/external/images/123") .build(); Constraints constraints = new Constraints.Builder() .setRequiresCharging(true) .setRequiredNetworkType(NetworkType.UNMETERED) .build(); OneTimeWorkRequest imageWorkRequest = new OneTimeWorkRequest.Builder(ImageProcessingWorker.class) .setInputData(inputData) .setConstraints(constraints) .build(); WorkManager.getInstance(context).enqueue(imageWorkRequest);
Benefits:
- Improves UI responsiveness by offloading intensive tasks
- Handles system constraints intelligently (battery, network)
- Survives app restarts for critical operations
- Manages task dependencies and chaining
Free tier: Part of Android Jetpack, free to use Documentation: WorkManager Documentation
8. Real-time Performance Monitoring
You can't improve what you don't measure. Implementing performance monitoring helps identify bottlenecks.
Implementation: New Relic Mobile API
New Relic provides comprehensive mobile app monitoring through their SDK.
Example integration for iOS:
// Initialize New Relic in AppDelegate func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { NewRelic.start(withApplicationToken: "YOUR_NEW_RELIC_TOKEN") return true } // Track custom interactions func loadProductCatalog() { NewRelic.startInteraction(withName: "Load Product Catalog") // Perform loading operation catalogService.loadProducts { products in // Store interaction identifier when complete NewRelic.stopCurrentInteraction() // Update UI self.displayProducts(products) } } // Record custom metrics func recordCheckoutPerformance(timeInMs: Double) { NewRelic.recordMetric( withName: "Checkout Time", category: "Shopping", value: timeInMs ) }
Postman request to query performance data:
GET https://api.newrelic.com/v2/mobile_applications/{app_id}/metrics/data.json
Headers:
X-Api-Key: {your_api_key}
Content-Type: application/json
Query Parameters:
names[]=Mobile/Crash
names[]=Mobile/HandledException
from=2023-05-01T00:00:00+00:00
to=2023-05-02T00:00:00+00:00
summarize=true
Benefits:
- Real-time monitoring of app performance
- Crash analysis and reporting
- User interaction tracking
- Network request monitoring
Free tier: Limited free tier with core features Documentation: New Relic Mobile Monitoring
Pre-caching for Lightning Fast App Startup
Predictive pre-caching loads resources before users need them, creating instantaneous experiences.
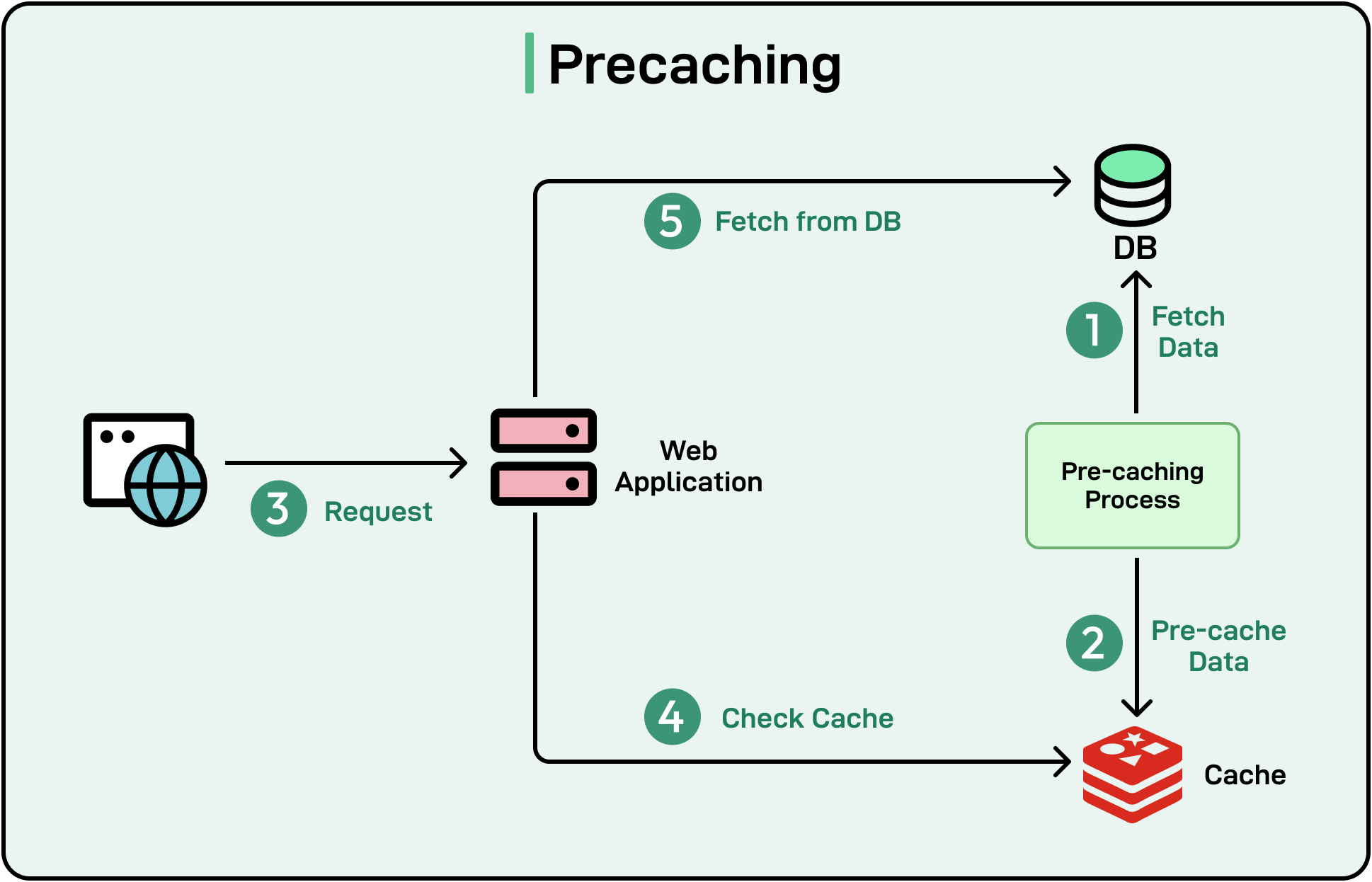
Implementation: Google's Prefetch API
Google provides a prefetch API for Android apps to load resources ahead of time.
Example implementation:
// In your Application class public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); // Configure prefetch PreloadConfig config = new PreloadConfig.Builder() .setMaxCacheSize(50 * 1024 * 1024) // 50MB .build(); Prefetcher.getInstance().initialize(this, config); } } // Prefetch content based on user behavior public void prefetchLikelyNextScreens() { // User is on product page, likely to check out next if (currentScreen == Screen.PRODUCT_DETAIL) { List<PrefetchUrlTask> urls = new ArrayList<>(); // Add checkout APIs to prefetch queue urls.add(new PrefetchUrlTask.Builder("https://api.example.com/checkout/info") .setPriority(PrefetchUrlTask.PRIORITY_HIGH) .build()); urls.add(new PrefetchUrlTask.Builder("https://api.example.com/payment/methods") .setPriority(PrefetchUrlTask.PRIORITY_MEDIUM) .build()); Prefetcher.getInstance().prefetchUrls(urls); } }
Benefits:
- Dramatically reduces perceived loading time
- Improves conversion rates at critical app moments
- Optimizes resource usage based on user behavior
- Works with existing networking libraries
Free tier: Part of Google Play services, free to use Documentation: Android Prefetch Documentation
Implementing a Comprehensive App Performance Strategy
For maximum performance gains, combine multiple optimization techniques:
- Start with measurement: Implement performance monitoring to identify bottlenecks
- Focus on critical paths: Optimize the most frequently used features first
- Layer optimizations: Apply multiple techniques to critical screens
- Test on real devices: Performance testing should include low-end devices
- Monitor continuously: Set up alerts for performance regressions
Example: E-commerce App Performance Overhaul
Here's a practical example combining multiple APIs to optimize a shopping app:
// 1. Configure CDN for static assets val appConfig = AppConfig.Builder() .setStaticAssetsDomain("cdn.fastly.yourapp.com") .build() // 2. Set up image optimization with Cloudinary val imageLoader = CloudinaryImageLoader.Builder(context) .setCloudName("your-cloud-name") .setImageQuality("auto:good") .setDefaultFormat("webp") .build() // 3. Initialize performance monitoring NewRelic.start(context, "your-new-relic-key") // 4. Configure caching strategy val cacheConfig = CacheConfig.Builder() .setProductCacheTtl(TimeUnit.HOURS.toMillis(1)) .setCategoryCacheTtl(TimeUnit.DAYS.toMillis(1)) .build() // 5. Set up prefetching for likely navigation paths class ShoppingApp : Application() { override fun onCreate() { super.onCreate() // Register navigation prediction model val navPredictor = NavigationPredictor.Builder() .addPrediction(Screen.PRODUCT_LIST, Screen.PRODUCT_DETAIL, 0.7f) .addPrediction(Screen.PRODUCT_DETAIL, Screen.CART, 0.5f) .addPrediction(Screen.CART, Screen.CHECKOUT, 0.9f) .build() // Configure prefetch based on predictions val prefetcher = Prefetcher.getInstance() prefetcher.initialize(this) prefetcher.setNavigationPredictor(navPredictor) } } // 6. Use background processing for heavy operations class ProductImageProcessor : Worker() { override fun doWork(): Result { // Process product images in the background return try { val images = inputData.getStringArray("IMAGE_URIS") ?: return Result.failure() processImages(images) Result.success() } catch (e: Exception) { NewRelic.recordError(e) Result.retry() } } }
Conclusion
App performance is no longer optional—it's a competitive necessity. By implementing the strategies and APIs outlined in this guide, you can significantly improve your app's speed, responsiveness, and user experience.
Remember that performance optimization is an ongoing process. User expectations continually rise, and what was fast yesterday may feel slow tomorrow. Maintain a performance budget, measure consistently, and make optimization a regular part of your development cycle.
The free and open APIs mentioned provide powerful tools to implement these optimizations without significant investment, making performance enhancement accessible to developers and companies of all sizes.
References
- Android Developers: App Performance Guide
- Apple Developer: Performance Best Practices
- Web.dev: Mobile Performance Optimization
- Firebase Documentation: Performance Monitoring
- Cloudinary Documentation: Mobile SDK
- Realm Documentation: Mobile Database
- New Relic Documentation: Mobile Monitoring
- Google Developers: Android Vitals
- WorkManager Documentation: Background Processing
- Fastly Documentation: Mobile SDK Integration